Note
Go to the end to download the full example code.
Basic selection rules example#
This example shows the basic usage of selection rules, which enable you to select elements through geometrical operations and thus to shape plies. The example only shows the PyACP part of the setup. For more advanced selection rule usage, see Advanced rules example. For a complete composite analysis, see Basic PyACP workflow example.
Import modules#
Import the standard library and third-party dependencies.
import pathlib
import tempfile
Import the PyACP dependencies.
from ansys.acp.core import ACPWorkflow, LinkedSelectionRule, launch_acp
from ansys.acp.core.example_helpers import ExampleKeys, get_example_file
Start ACP and load the model#
Get the example file from the server.
tempdir = tempfile.TemporaryDirectory()
WORKING_DIR = pathlib.Path(tempdir.name)
input_file = get_example_file(ExampleKeys.MINIMAL_FLAT_PLATE, WORKING_DIR)
Launch the PyACP server and connect to it.
acp = launch_acp()
Define the input file and instantiate an ACPWorkflow
instance.
The ACPWorkflow
class provides convenience methods that simplify file handling.
It automatically creates a model based on the input file.
This example’s input file contains a flat plate with a single ply.
workflow = ACPWorkflow.from_acph5_file(
acp=acp,
acph5_file_path=input_file,
local_working_directory=WORKING_DIR,
)
model = workflow.model
print(workflow.working_directory.path)
print(model.unit_system)
/tmp/tmphzdhh9ea
mks
Visualize the loaded mesh.
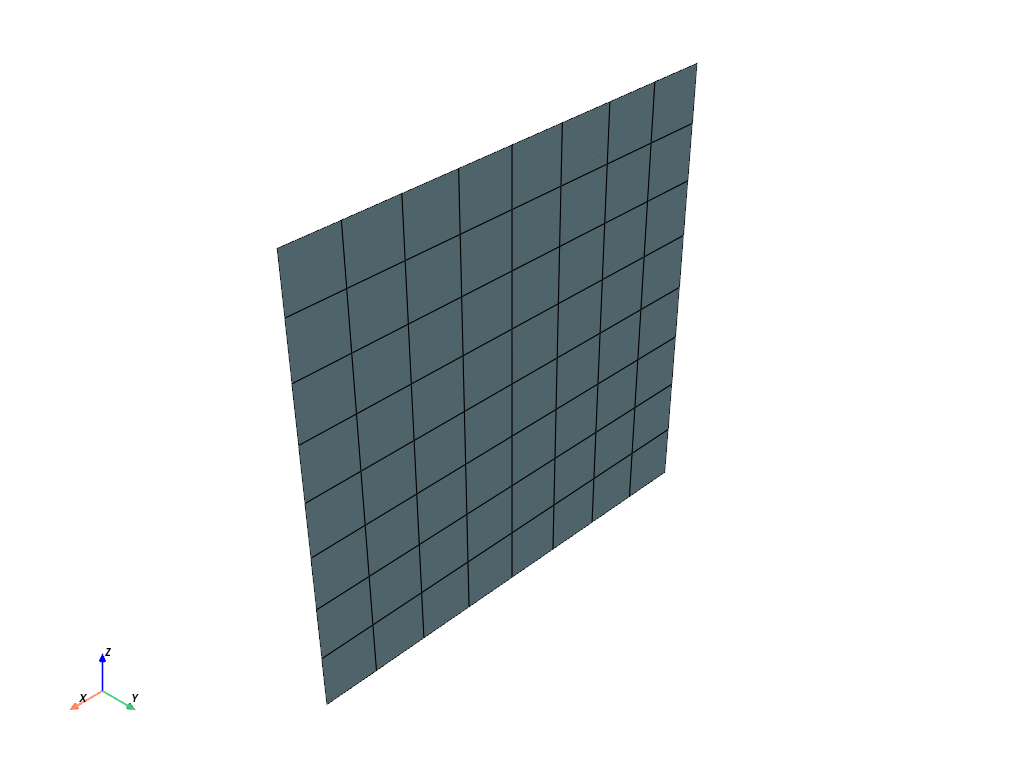
Create a Parallel Rule#
Create parallel selection rule and assign it to the existing ply.
parallel_rule = model.create_parallel_selection_rule(
name="parallel_rule",
origin=(0, 0, 0),
direction=(1, 0, 0),
lower_limit=0.005,
upper_limit=1,
)
modeling_ply = model.modeling_groups["modeling_group"].modeling_plies["ply"]
modeling_ply.selection_rules = [LinkedSelectionRule(parallel_rule)]
Plot the ply thickness.
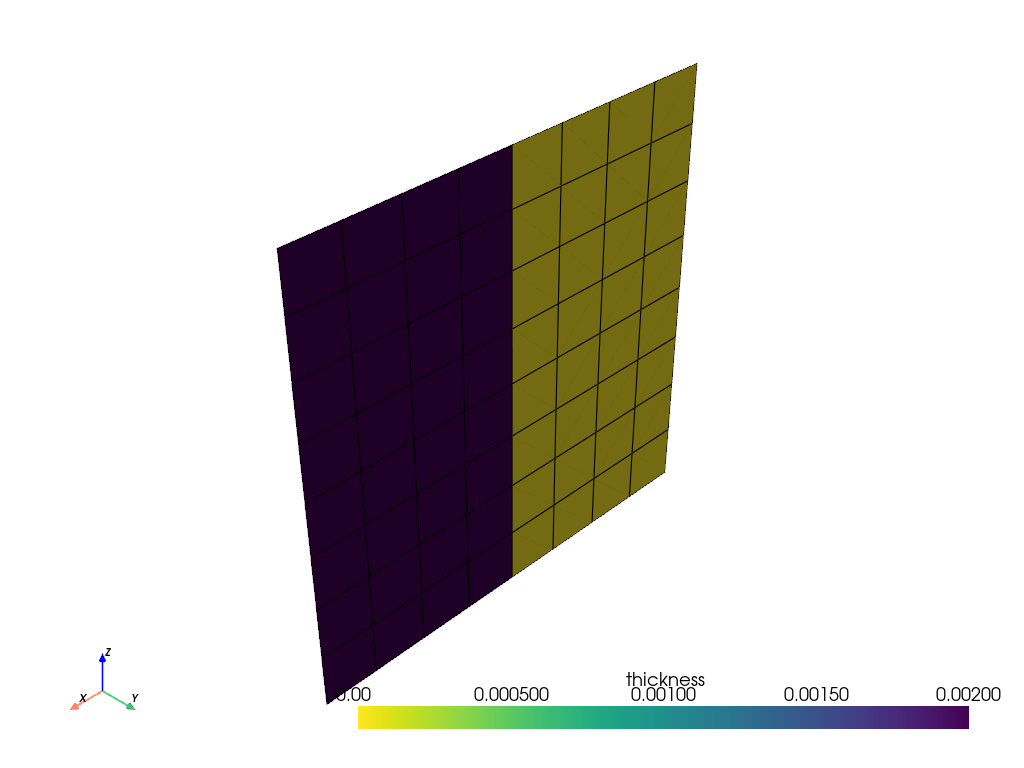
Create a Cylindrical Rule#
Create a cylindrical selection rule and add it to the ply. This will intersect the two rules.
cylindrical_rule = model.create_cylindrical_selection_rule(
name="cylindrical_rule",
origin=(0.005, 0, 0.005),
direction=(0, 1, 0),
radius=0.002,
)
modeling_ply.selection_rules.append(LinkedSelectionRule(cylindrical_rule))
Plot the ply thickness.
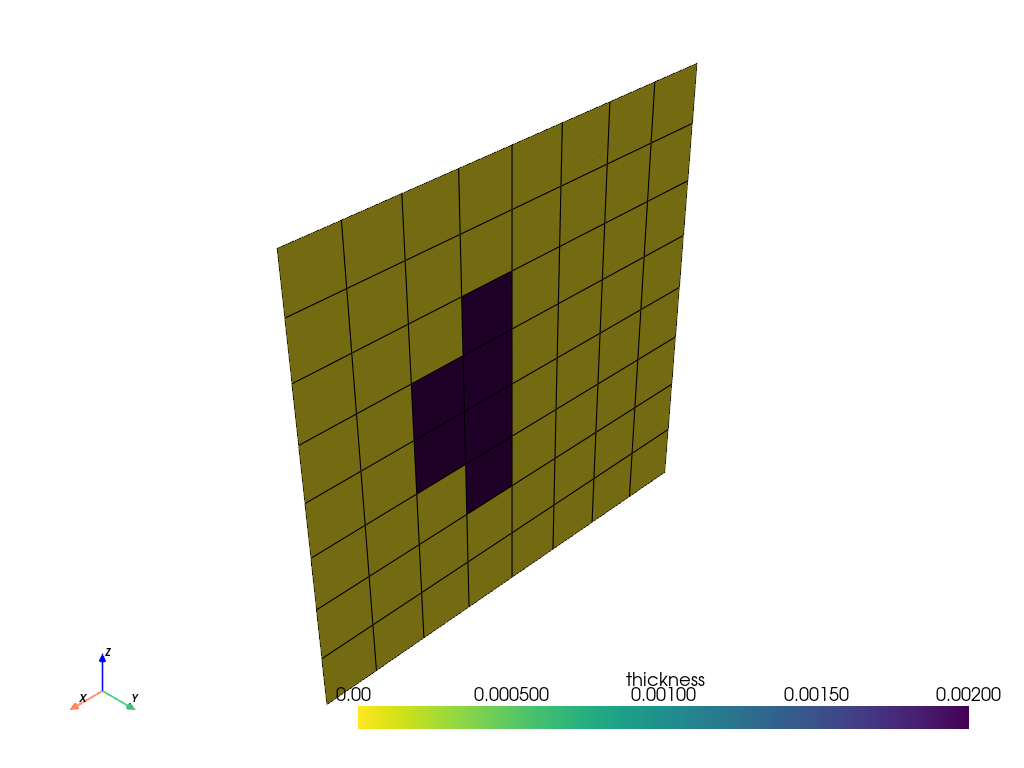
Create a Spherical Rule#
Create a spherical selection rule and assign it to the ply. Now, only the spherical rule is active.
spherical_rule = model.create_spherical_selection_rule(
name="spherical_rule",
origin=(0.003, 0, 0.005),
radius=0.002,
)
modeling_ply.selection_rules = [LinkedSelectionRule(spherical_rule)]
Plot the ply thickness.
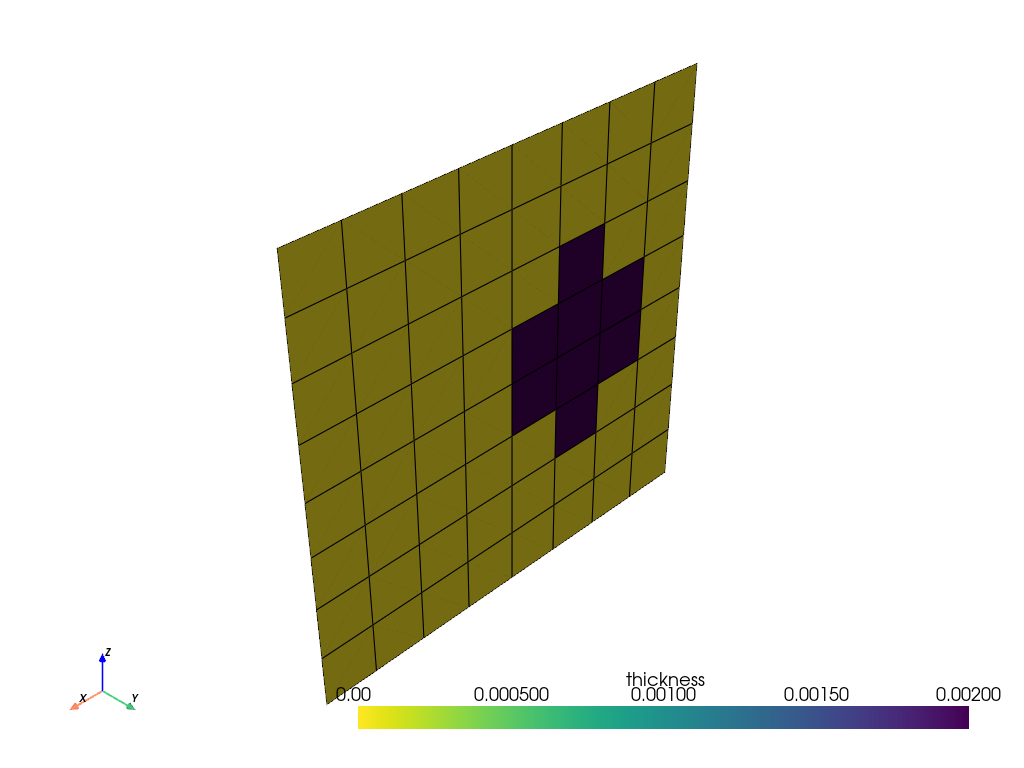
Create a Tube Rule#
Create a tube selection rule and assign it to the ply. Now, only the tube rule is active.
tube_rule = model.create_tube_selection_rule(
name="spherical_rule",
# Select the pre-exsting _FIXEDSU edge which is the edge at x=0
edge_set=model.edge_sets["_FIXEDSU"],
inner_radius=0.001,
outer_radius=0.003,
)
modeling_ply.selection_rules = [LinkedSelectionRule(tube_rule)]
Plot the ply thickness.
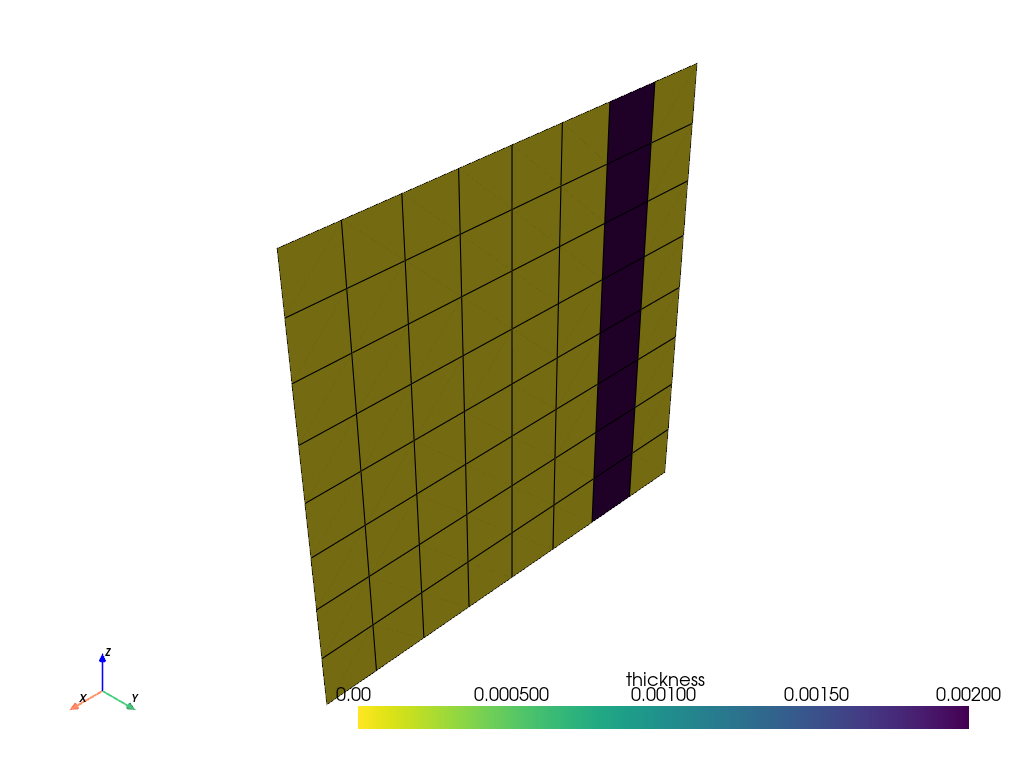
Total running time of the script: (0 minutes 5.912 seconds)