Note
Go to the end to download the full example code.
Sensor#
The Sensor
capabilities to analyze the composite structure
is demonstrated in this example. A sensor is used to compute the weight,
area, cost, etc. of the model or specific entities such as ply material,
modeling ply, etc.
Import modules#
Import the standard library and third-party dependencies.
import pathlib
import tempfile
import pyvista
Import the PyACP dependencies.
from ansys.acp.core import SensorType, UnitSystemType, launch_acp
from ansys.acp.core.extras import (
RACE_CARE_NOSE_CAMERA_METER,
ExampleKeys,
get_example_file,
set_plot_theme,
)
Set the plot theme for the example. This is optional, and ensures that you get the same plot style (theme, color map, etc.) as in the online documentation.
set_plot_theme()
Start ACP and load the model#
Get the example file from the server.
tempdir = tempfile.TemporaryDirectory()
WORKING_DIR = pathlib.Path(tempdir.name)
acph5_input_file = get_example_file(ExampleKeys.RACE_CAR_NOSE_ACPH5, WORKING_DIR)
Launch the PyACP server and connect to it.
acp = launch_acp()
Load the model from the input file which contains a formula 1 front wing with layup.
model = acp.import_model(acph5_input_file)
model.unit_system = UnitSystemType.SI
print(model.unit_system)
model.update()
si
The plot shows the total laminate thickness per element.
thickness_data = model.elemental_data.thickness
if thickness_data is not None:
plotter = pyvista.Plotter()
plotter.add_mesh(thickness_data.get_pyvista_mesh(model.mesh), show_edges=False)
plotter.camera_position = RACE_CARE_NOSE_CAMERA_METER
plotter.show()
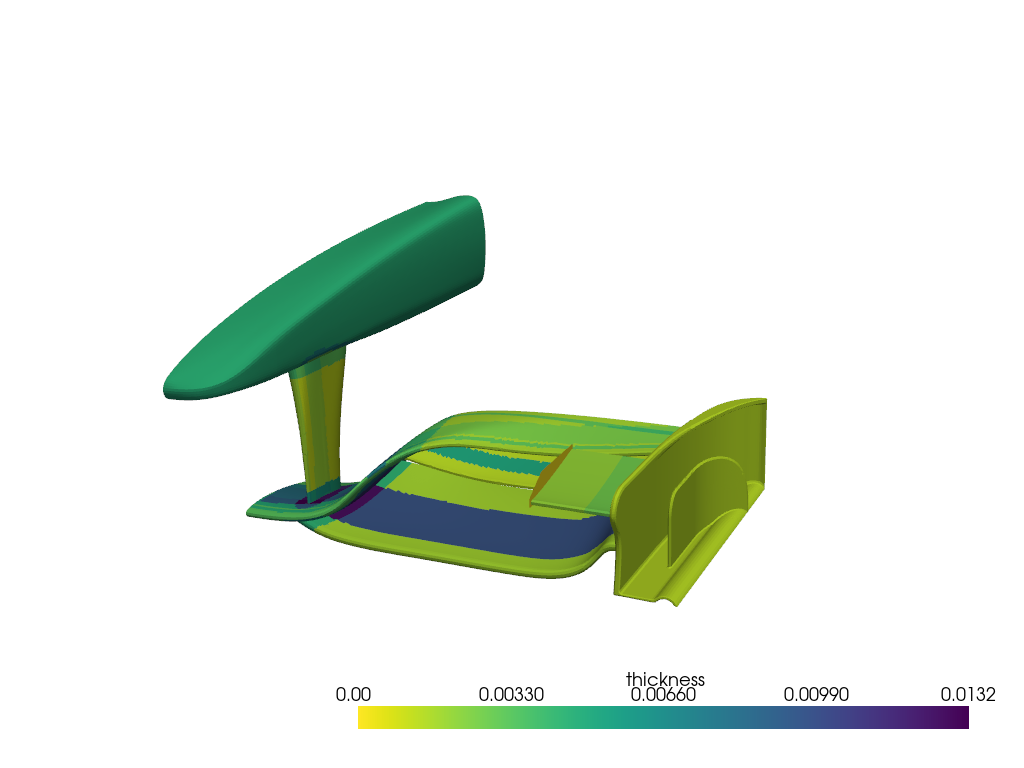
Set price per area for all fabrics.
model.fabrics["UD"].area_price = 15 # $/m^2
model.fabrics["woven"].area_price = 23 # $/m^2
model.fabrics["core_4mm"].area_price = 7 # $/m^2
Sensor by area#
Entire Model#
The first sensor is applied to the entire model to compute for example the total weight, area of production material, and material cost.
sensor_by_area = model.create_sensor(
name="By Area",
sensor_type=SensorType.SENSOR_BY_AREA,
entities=[model.element_sets["All_Elements"]],
)
Update the model to compute the sensor values.
model.update()
def print_measures(my_sensor):
if my_sensor.price is not None:
print(f"Price: {my_sensor.price:.2f} $")
if my_sensor.weight is not None:
print(f"Weight: {my_sensor.weight:.2f} kg")
if my_sensor.covered_area is not None:
print(f"Covered area: {my_sensor.covered_area:.2f} m²")
if my_sensor.modeling_ply_area is not None:
print(f"Modeling ply area: {my_sensor.modeling_ply_area:.2f} m²")
if my_sensor.production_ply_area is not None:
print(f"Production ply area: {my_sensor.production_ply_area:.2f} m²")
cog = my_sensor.center_of_gravity
if cog is not None:
print(f"Center of gravity: ({cog[0]:.2f}, {cog[1]:.2f}, {cog[2]:.2f}) m")
Print the values. The production ply area
is the area of production material.
print_measures(sensor_by_area)
Price: 208.84 $
Weight: 4.48 kg
Covered area: 1.70 m²
Modeling ply area: 5.31 m²
Production ply area: 11.04 m²
Center of gravity: (0.42, 0.10, -0.09) m
Scope to a specific component#
Compute the measures for the nose only. Note that OrientedSelectionSet
can also be used to scope the sensor.
eset_nose = model.element_sets["els_nose"]
sensor_by_area.entities = [eset_nose]
model.update()
print_measures(sensor_by_area)
plotter = pyvista.Plotter()
plotter.add_mesh(eset_nose.mesh.to_pyvista(), show_edges=False, opacity=1, color="turquoise")
plotter.add_mesh(model.mesh.to_pyvista(), show_edges=False, opacity=0.2)
plotter.camera_position = RACE_CARE_NOSE_CAMERA_METER
plotter.show()
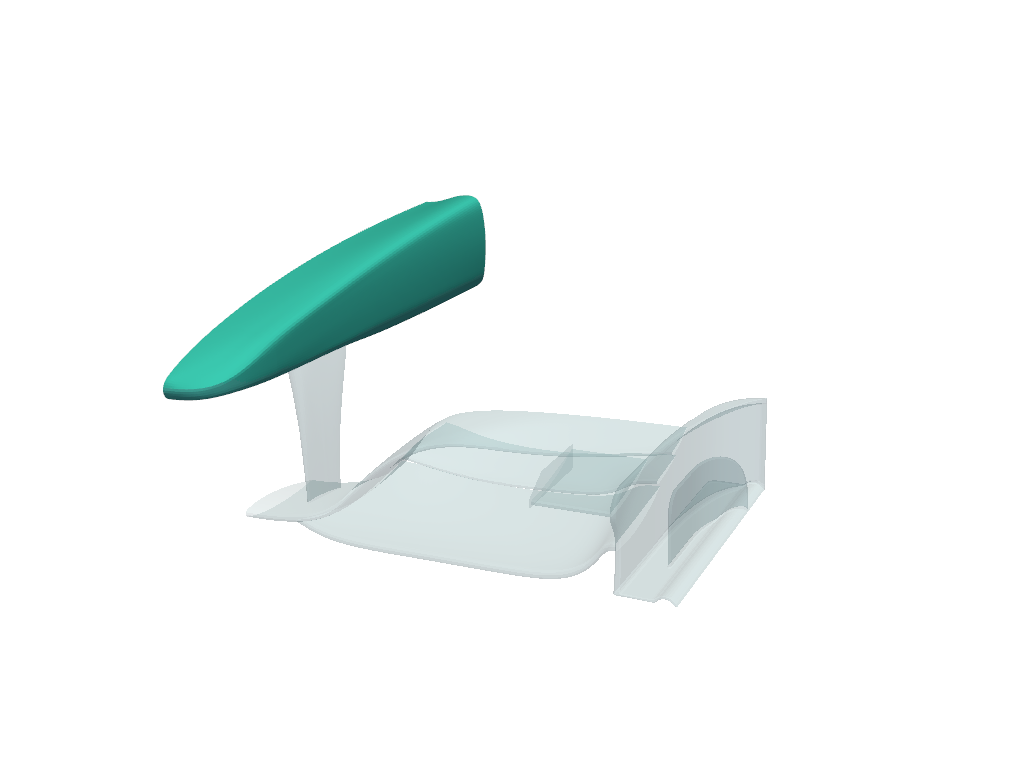
Price: 25.21 $
Weight: 0.56 kg
Covered area: 0.39 m²
Modeling ply area: 1.18 m²
Production ply area: 1.64 m²
Center of gravity: (0.09, 0.37, -0.22) m
Sensor by material#
A sensor can also be used to compute the amount of a certain ply material
(Fabric
, Stackup
, SubLaminate
).
sensor_by_material = model.create_sensor(
name="By Material",
sensor_type=SensorType.SENSOR_BY_MATERIAL,
entities=[model.fabrics["UD"]],
)
print_measures(sensor_by_area)
Price: 25.21 $
Weight: 0.56 kg
Covered area: 0.39 m²
Modeling ply area: 1.18 m²
Production ply area: 1.64 m²
Center of gravity: (0.09, 0.37, -0.22) m
Sensor by ply#
A sensor can also be scoped to a specific ply or a list of plies. In this example, a ply of the suction side and a ply of the pressure side of wing 3 are selected.
mg = model.modeling_groups["wing_3"]
modeling_plies = [
mg.modeling_plies["mp.wing_3.1_suction"],
mg.modeling_plies["mp.wing_3.1_pressure.2"],
]
sensor_by_ply = model.create_sensor(
name="By Ply",
sensor_type=SensorType.SENSOR_BY_PLIES,
entities=modeling_plies,
)
model.update()
print_measures(sensor_by_ply)
plotter = pyvista.Plotter()
for ply in modeling_plies:
plotter.add_mesh(ply.mesh.to_pyvista(), show_edges=False, opacity=1, color="turquoise")
plotter.add_mesh(model.mesh.to_pyvista(), show_edges=False, opacity=0.2)
plotter.camera_position = RACE_CARE_NOSE_CAMERA_METER
plotter.show()
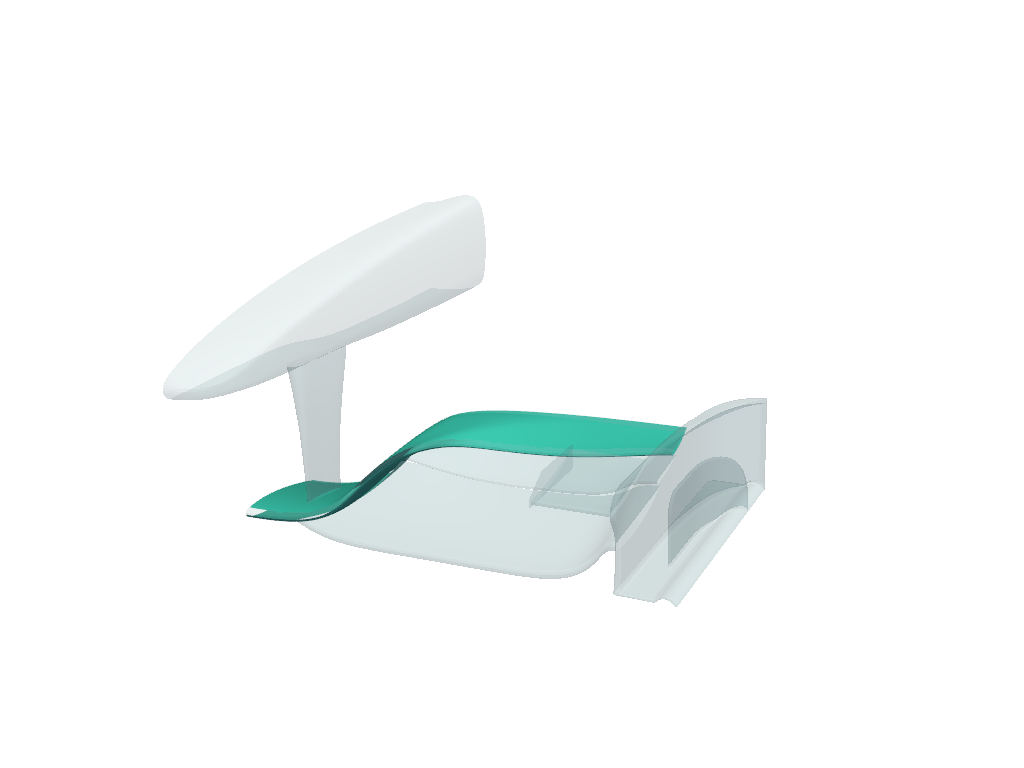
Price: 10.86 $
Weight: 0.23 kg
Covered area: 0.24 m²
Modeling ply area: 0.24 m²
Production ply area: 0.47 m²
Center of gravity: (0.30, 0.08, -0.10) m
Sensor by solid model#
Note
The sensor by solid model is only supported in PyACP from server version 25.2 onwards.
Finally, a sensor can be scoped to a solid model. In this case, only the weight and center of gravity are computed.
solid_model = model.create_solid_model()
solid_model.element_sets = [model.element_sets["els_nose"]]
solid_model.drop_off_material = model.materials["Resin_Epoxy"]
sensor_by_solid_model = model.create_sensor(
name="By Solid Model",
sensor_type=SensorType.SENSOR_BY_SOLID_MODEL,
entities=[solid_model],
)
model.update()
print_measures(sensor_by_solid_model)
model.solid_mesh.to_pyvista().plot(show_edges=True)
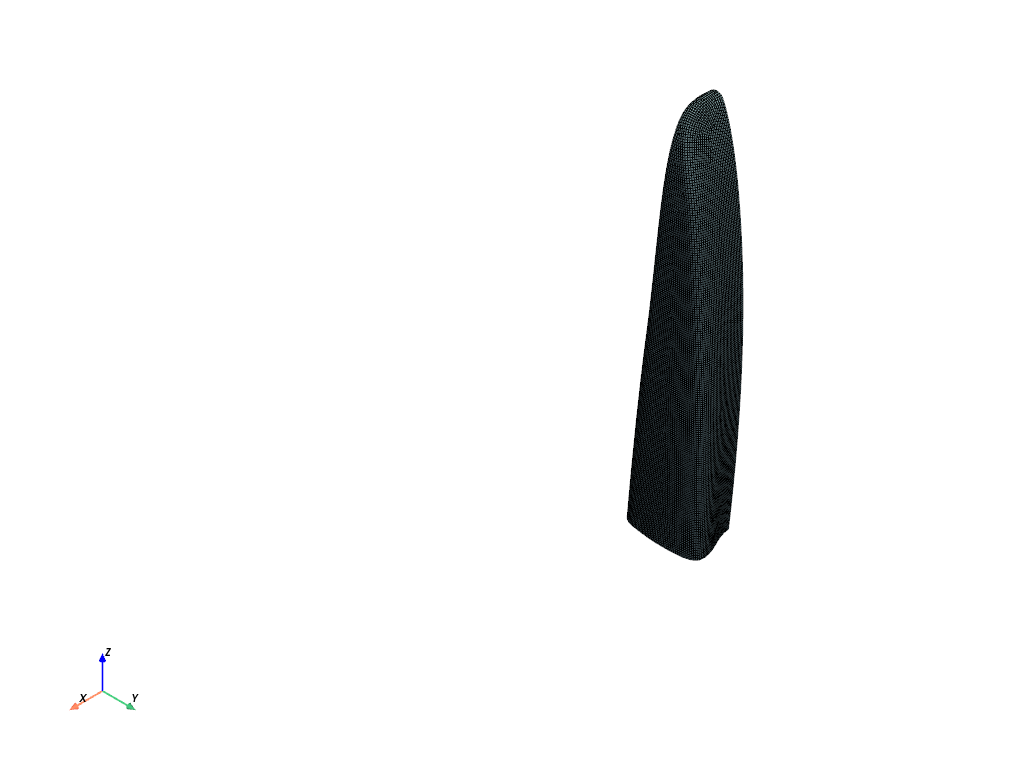
Weight: 0.55 kg
Center of gravity: (0.09, 0.37, -0.22) m
Total running time of the script: (0 minutes 32.224 seconds)